In Microsoft Dynamics 365 F&O we can show data(editable) on a form that is not directly in that data source of the form but is linked to that data source somehow. In this case instead of adding another data source we can just add a edit method on the form level or table level.
Also in some scenarios, data is dynamic which means it is not stored in any table and we need to generate it dynamically. In that case, we create a edit method on the table level or form level. so basically we can create a edit method on the table level and form level. Usability decreases when creating a edit method on form since the scope of that edit method is within that form only. but in the case of a table usability is better since the scope of that edit method is in all the forms of that table.
Limitation of D365 Edit method:
1. We can’t filter data in the edit method field.
2. Data generates dynamically hence it puts a load on the form.
Benefits of the D365 Edit method:
Gets user-expected data no matter the complexity between the relation of and tables.
D365 Edit method can be used in any of the following:
- Real
- String
- Logical
1. Example of Real in D365 Edit method: (This is not a real case scenario, just imaginary scenario)
public class LSCustomTable extends common
{
/// <summary>
/// Sets A and B to half of _c if 'set' is true; otherwise, returns the sum of A and B.
/// </summary>
/// <param name="set">Boolean indicating whether to set or retrieve values.</param>
/// <param name="_c">The input value used when setting A and B.</param>
/// <returns>Returns the sum of A and B if 'set' is false.</returns>
[SysClientCacheDataMethodAttribute(true)]
public edit real editC(boolean set, real _c)
{
real C;
if (set)
{
ttsbegin;
this.A = _c/2;
this.B = _c/2;
ttscommit;
}
else
{
C = this.A + this.B;
}
return C;
}
}
2. Example of String in D365 Edit method: (This is not a real case scenario, just imaginary scenario)
public class LSCustomTable extends common
{
/// <summary>
/// Sets A and B to the value of _c if 'set' is true; otherwise, returns the concat of A and B
/// </summary>
/// <param name="set">Boolean indicating whether to set or retrieve values.</param>
/// <param name="_c">The input value (string) used when setting A and B.</param>
/// <returns>Returns the concatenation of A and B as a string if 'set' is false.</returns>
[SysClientCacheDataMethodAttribute(true)]
public edit str editC(boolean set, str _c)
{
str result;
if (set)
{
ttsbegin;
this.A = _c; // Set A to _c
this.B = _c; // Set B to _c
ttscommit;
}
else
{
// Concatenate A and B with a space (or any delimiter you prefer)
result = this.A + " " + this.B;
}
return result;
}
}
3. Example of Logical operation in D365 Edit method: (This is not a real case scenario, just imaginary scenario)
public class LSCustomTable extends common
{
/// <summary>
/// Sets the values of A and B to the input _c if 'set' is true.
/// If 'set' is false, returns the concatenation of A and B.
/// </summary>
/// <param name="set">Boolean indicating whether to set or retrieve values.</param>
/// <param name="_c">The input value (string) used when setting A and B.</param>
/// <returns>Returns the concatenation of A and B as a string if 'set' is false.</returns>
[SysClientCacheDataMethodAttribute(true)]
public edit str editC(boolean set, str _c)
{
str result;
if (set)
{
// Basic validation for the input string
if (!_c || strlen(_c) == 0)
{
throw error("Input value _c cannot be empty.");
}
ttsbegin;
this.A = _c; // Set field A to _c
this.B = _c; // Set field B to _c
ttscommit;
}
else
{
// Validate that fields A and B are not empty before concatenating
if (this.A && this.B)
{
result = strFmt("%1 - %2", this.A, this.B); // Concatenate A and B with a hyphen
}
else
{
result = "A or B has not been set.";
}
}
return result;
}
}
Once this is done just apply the method on the Data method property of the form control as shown below
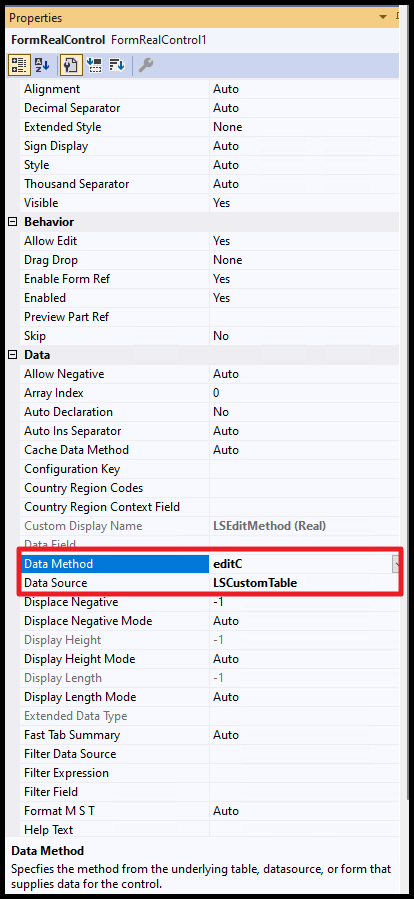
How to Cache D365 Edit method:
We can also cache a edit method so that load on DB is one time, only when the form is loaded first time which makes the performance of the form better.
[SysClientCacheDataMethodAttribute(true)]
We just need to add the above code, above the d365 edit method.
You can also check out my previous blog: Create a custom service in d365 FO
Need help? Connect Atul
- D365 Data entitiy Insert method COC - October 30, 2024
- D365 Joins - October 16, 2024
- D365 Find method and Exist method - October 9, 2024