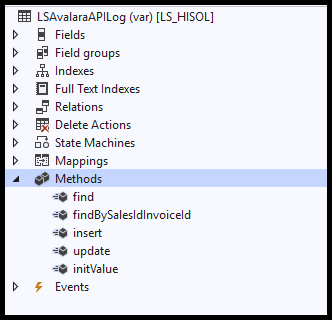
Table methods are the base level of code that can be done for validation. The code in methods is also referred to as custom business logic. When records are inserted, deleted, updated, or validated from a table various standard methods are called.
We can alter the standard methods by overriding the standard methods. or creating COC for the table.
Below are a few examples of the most commonly used Table methods:
1. InitValue() method:
If we create a new record from form or table level, this method is called. It is used to set a default value for specific fields.
public void initValue()
{
super();
this.custName = "Dynamics Community 101";
}
Due to this method, the field custName will now have the default value “Atul”.
2. ModifiedField() method:
Whenever the value of a field is changed/modified this method is called upon. It is used to perform certain actions when we modify certain fields.
public void modifiedField(fieldId _fieldId)
{
switch(_fieldId)
{
case fieldnum(custTable, custName):
this.custId="15";
break;
default:
super(_fieldId);
}
}
Whenever the customer name of an existing record or new record is modified, then you will notice that custID is immediately set to “15”.
3. Orig() method:
When the value of a field is modified, it is possible to recall the previous value of that field by using below code.
this.orig().salesId;
4. ValidateField() method:
This method is used for validation and has a boolean return type. If it returns false, the application will prevent the user from making any changes in that field.
public boolean validateField(fieldId _fieldIdToCheck)
{
boolean ret;
ret = super(_fieldIdToCheck);
if (ret)
{
switch (_fieldIdToCheck)
{
case fieldnum(custTable, custName):
if (strlen(this.custName
) <= 3)
ret = checkFailed("Customer name must be greater than 3 characters.");
}
}
return ret;
}
5. ValidateWrite() method:
This method is used to validate each mandatory field. It gives back data of boolean type. If it returns false then the insert operation will not happen.
public boolean validateWrite()
{
boolean ret;
if(this.custName != "")
ret = super();
else
warning(" Please fill the value");
return ret;
}
6. ValidateDelete() method:
When a user tries to delete a record then this method is first called. Only if this returns true then the record will be deleted,
public boolean validateDelete()
{
boolean ret;
ret = super();
info(this.custName);
return ret;
}
7. Insert() and Update() method:
These are standard methods that are called while inserting or updating a record.
public void Insert()
{
custTable custTable
;
ttsbegin;
.initValue();custTable
.custId = "101";custTable
.custName = "DynamcisCommunity101";custTable
.custBalance = "101";custTable
if (
.validateWrite())custTable
.insert();custTable
ttscommit;
}
Need help? Connect Atul
- D365 Data entitiy Insert method COC - October 30, 2024
- D365 Joins - October 16, 2024
- D365 Find method and Exist method - October 9, 2024