In this article, we will see how to Create a custom service in d365 FO
Custom service endpoint overview:
You can Create a custom service in d365 FO. When we write a custom service under a service group, the service group is always deployed on 2 endpoints:
1. SOAP endpoint to Create a custom service in d365 FO
Example endpoint for a dev environment:
https://DEVURL.cloud.onebox.dynamics.com/soap/services/UserSessionService?wsdl
Example endpoint for a non-dev environment:
https:///soap/services/UserSessionService?wsdl
2. JSON endpoint to Create a custom service in d365 FO
Example endpoint for a dev/Non dev environment
https://URL/api/services/service_group_name/service_name/operation_name
Now we will see how to create a custom service in d365 FO:
To create a custom service in d365 FO we will create 3 classes and 1 AOT service and 1 AOT service group,
1. Request class – In this class we will define the structure of request payload.
2. Response class – In this class we will define the response structure.
3. Service class – In this class we will write the logic.
4. Service – This is an AOT element where we will put the Service class name and method name.
5. Service Group – This is also an AOT element where we will put AOT service.
Request Class:
/// <summary>
/// DC Request is request contract class for DC Service
/// </summary>
[DataContractAttribute]
class DCRequest
{
private str salesId;
/// <summary>
/// parmsalesId is a parm method to get request of the sales order id in request of DC Service
/// </summary>
/// <param name = "_value">Sales order id</param>
/// <returns>Sales order id</returns>
[DataMember("salesId")]
public str parmsalesId(str _value = salesId)
{
if (!prmIsDefault(_value))
{
salesId = _value;
}
return salesId;
}
}
Response Class:
/// <summary>
/// DC Response is response contract class for DC Service
/// </summary>
[DataContractAttribute]
class DCResponse
{
private str custAccount;
/// <summary>
/// parmcustAccount is a parm method to return the customer account in reponse of DC Service
/// </summary>
/// <param name = "_value">Customer account</param>
/// <returns>Customer account</returns>
[DataMember("custAccount")]
public str parmcustAccount(str _value = custAccount)
{
if (!prmIsDefault(_value))
{
custAccount = _value;
}
return custAccount;
}
}
Service Class:
using Microsoft.DynamicsOnline.Infrastructure.Components.SharedServiceUnitStorage;
using System.IO;
/// <summary>
/// DCService is a class for custom service which will be used in AOT Service
/// </summary>
public class DCService
{
/// <summary>
/// Create method for getting the customer account from sales order
/// </summary>
/// <param name = "_request">Request class to get the sales Id</param>
/// <returns>Return class to send the customer Id in response</returns>
public DCResponse create(DCRequest _request)
{
salestable salestableLocal;
SalesId salesIdLocal = _request.parmsalesId();
var response = new DCResponse();
select firstonly crosscompany CustAccount from salestableLocal
where salestableLocal.SalesId == salesIdLocal;
response.parmcustAccount(strFmt("Customer account for sales id %1 is %2",_request.parmsalesId(),salestableLocal.CustAccount));
return response;
}
}
Once all the classes are created, now create a service, in my case I have named it as DCService
Now put the class property as DCService and Description as DCService and external name as DCService, as shown below
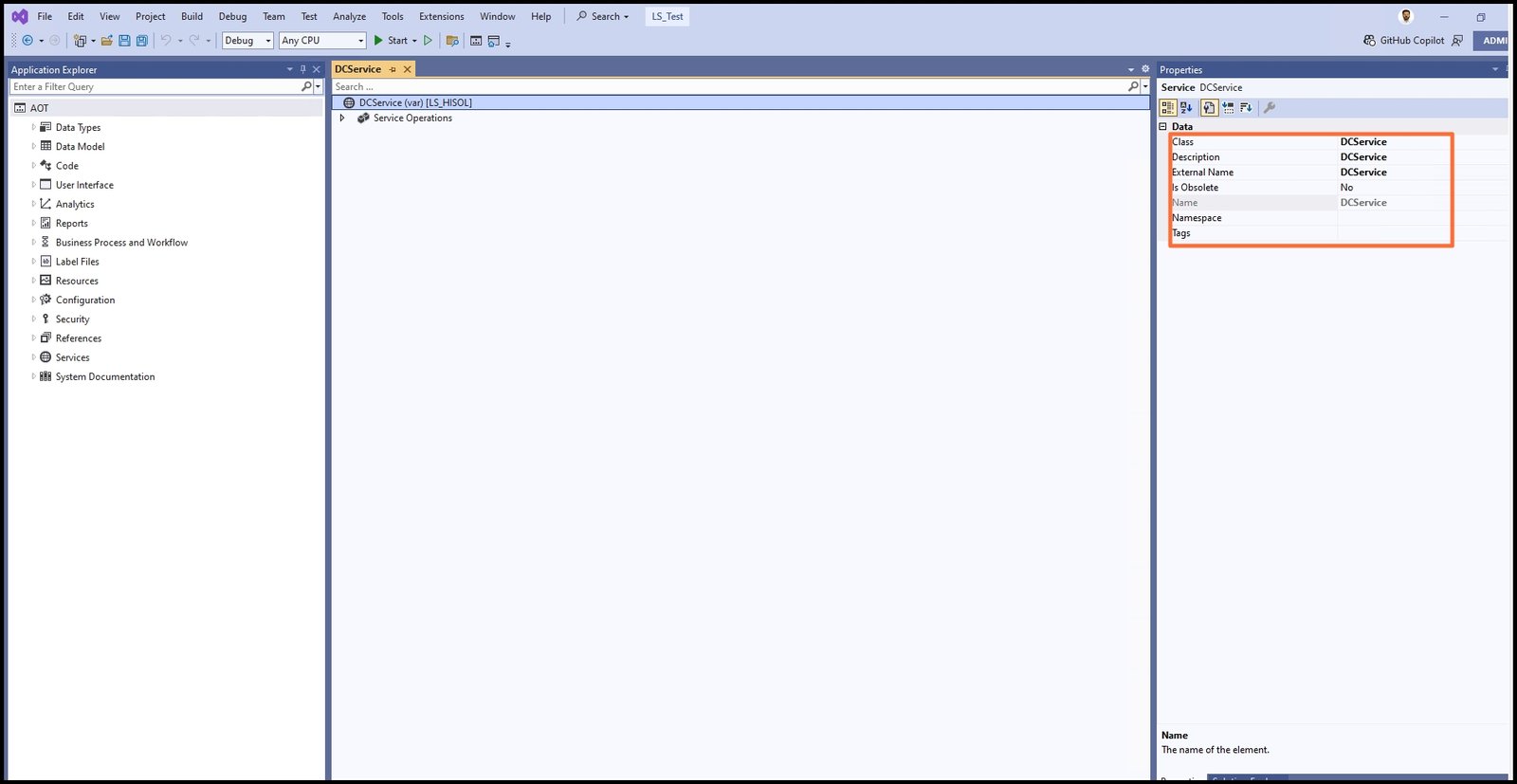
Now create a new service operation and fill in the method and name as shown below, in my case service operation name is ‘create’ and method is ‘create’
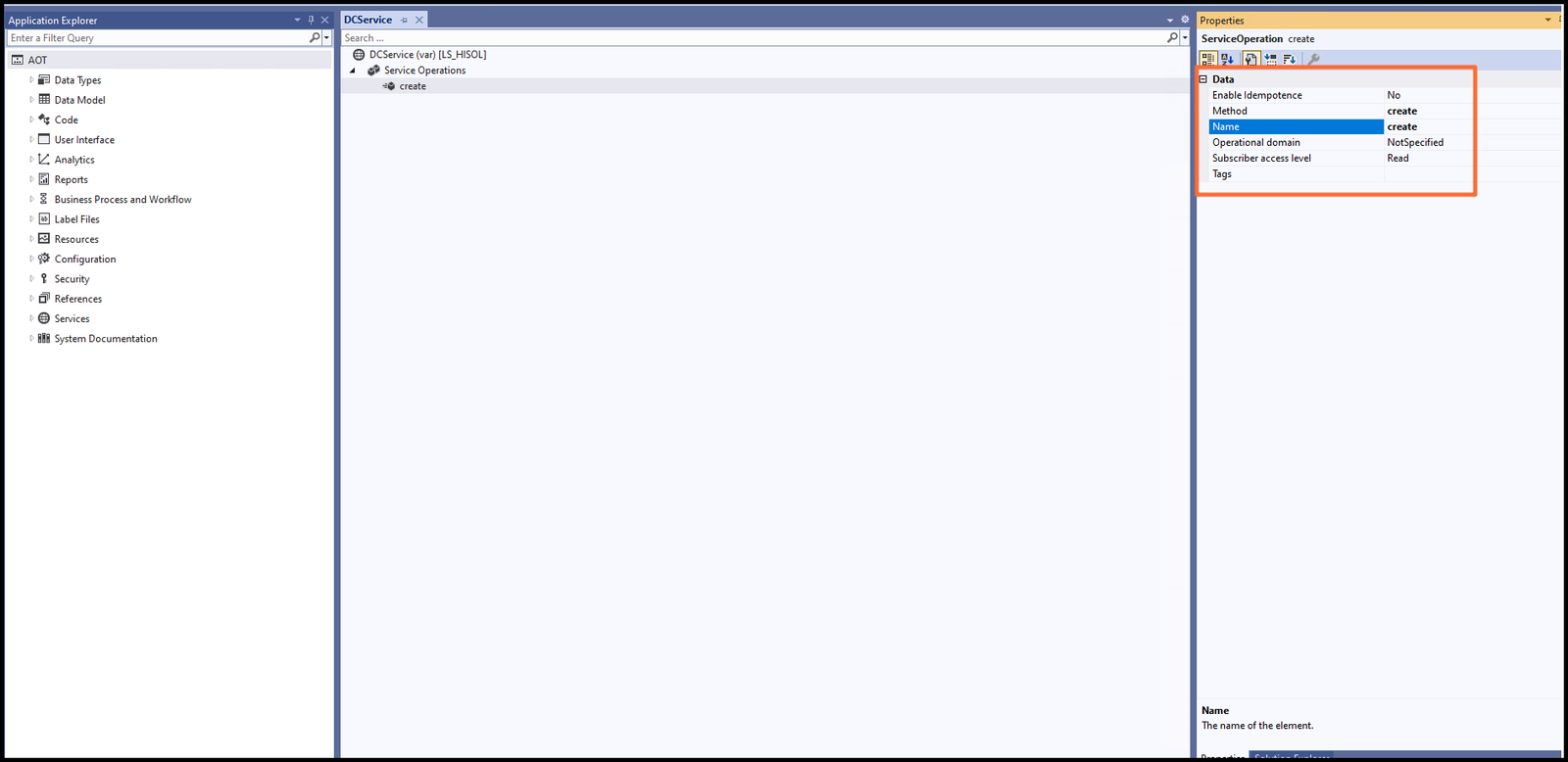
Now create a service group and make the auto deploy property as Yes,
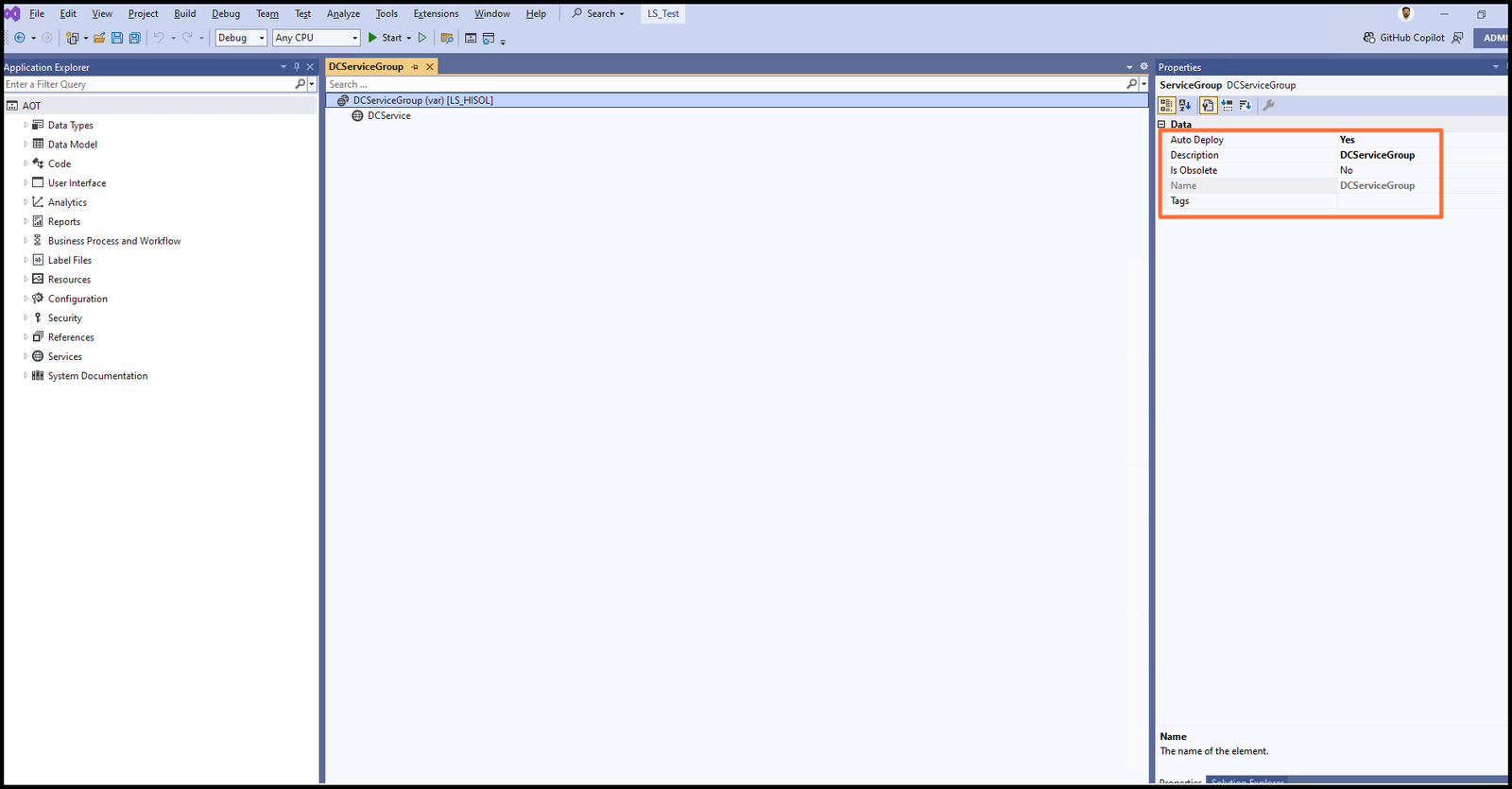
Now create a service in the service group and put the name as DCService and Service as DCService.
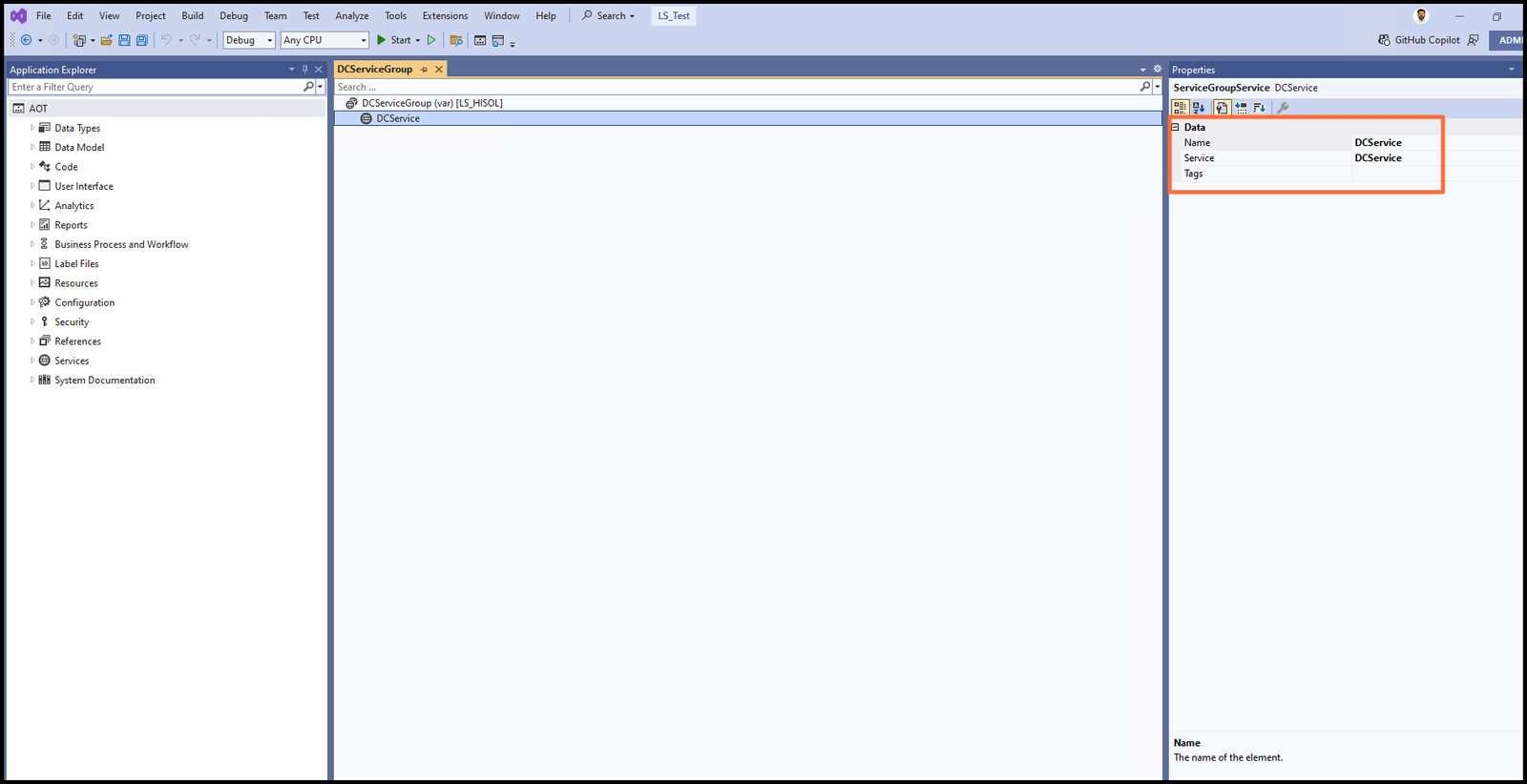
Once all this is done just build and synchronize the project area.
Now we will setup postman to call d365 custom service :
Before opening postman, we need to do app registration and take Application (client) ID, Client secrets, and Tenant ID.
Register App in Azure portal:
Open the Azure portal Link, click on Microsoft Entra ID as shown below
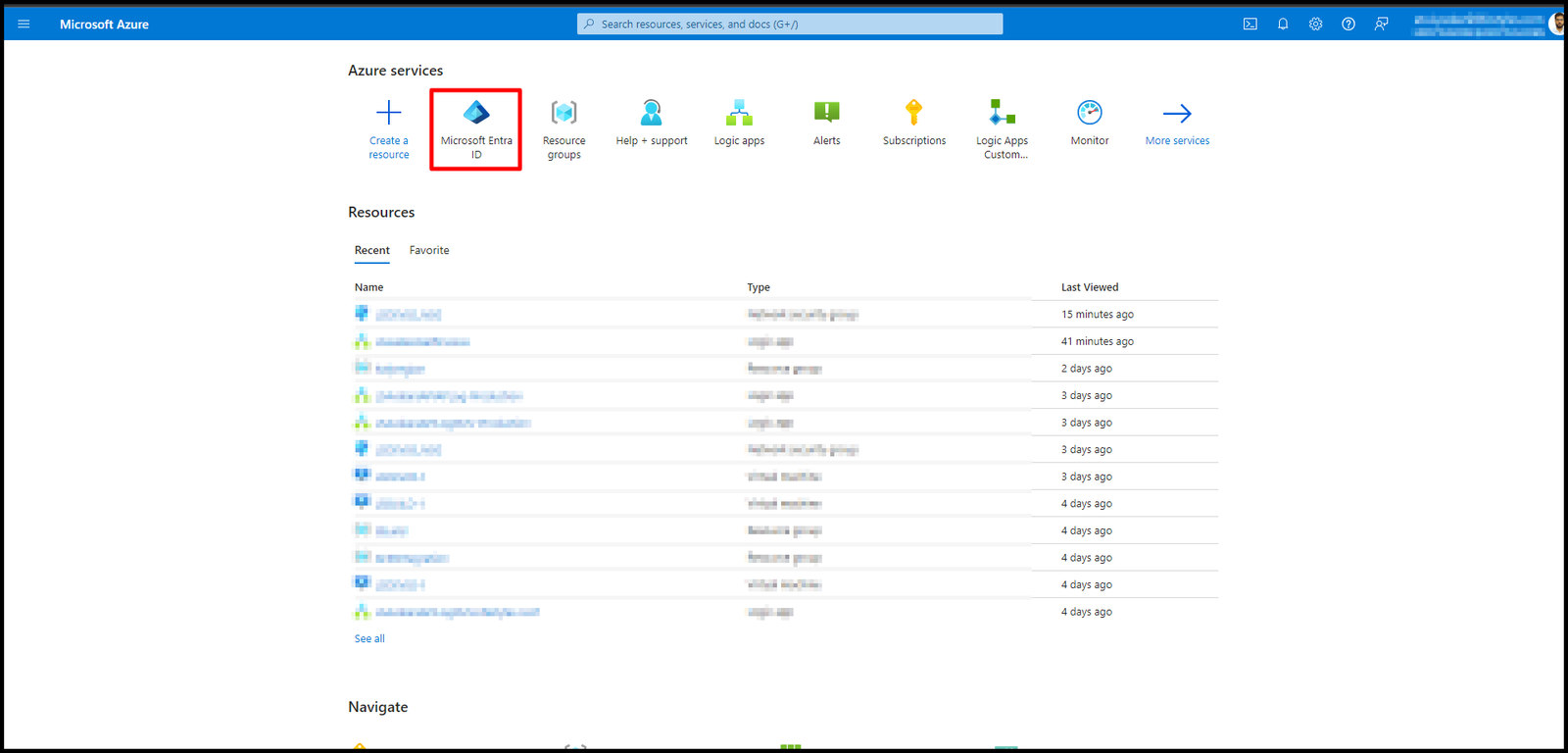
Now open App registration, as shown below
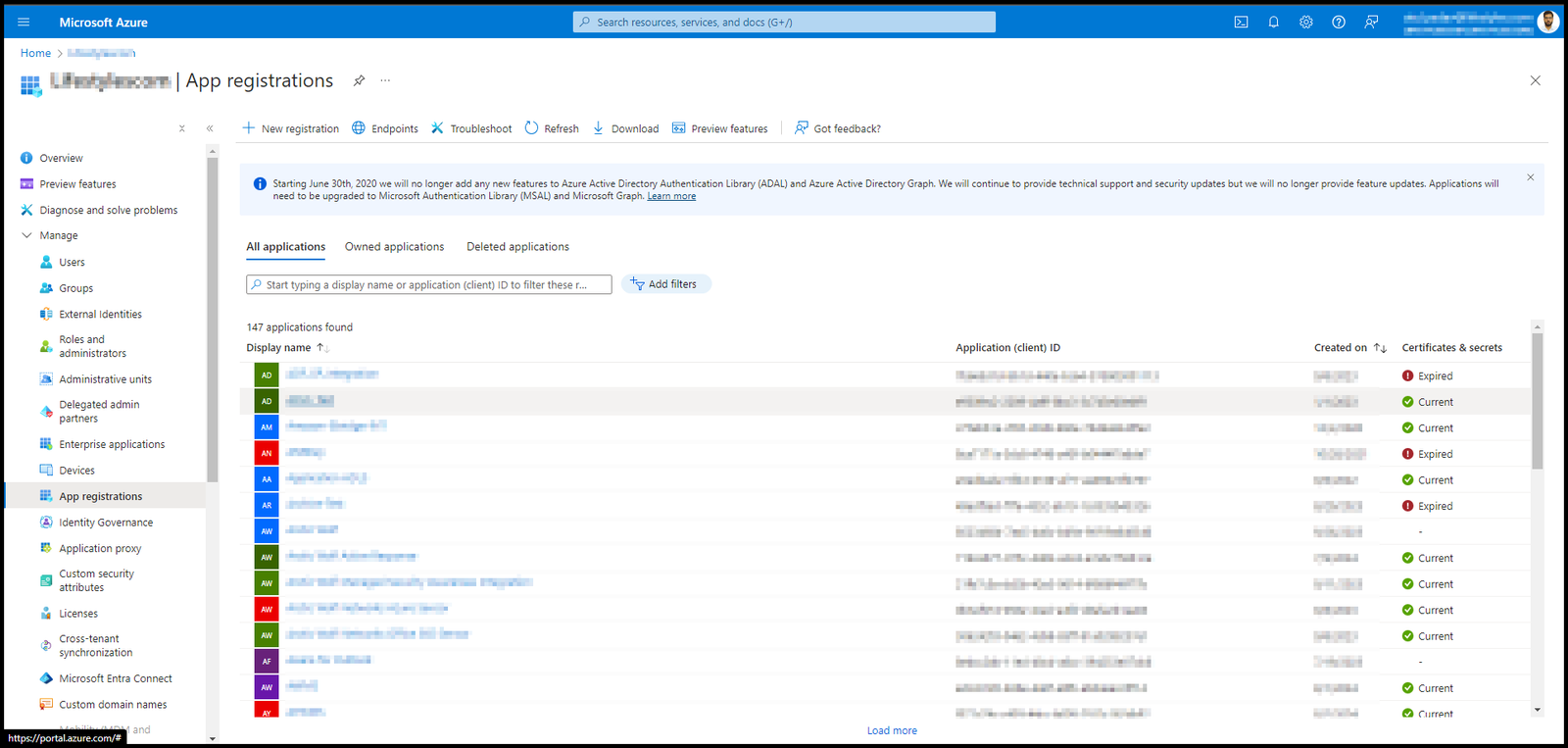
Now create a new App and note down the client ID, Tenant ID, and Client Secret. Once all done.
Make sure to add this client ID reference in the D365 as shown below
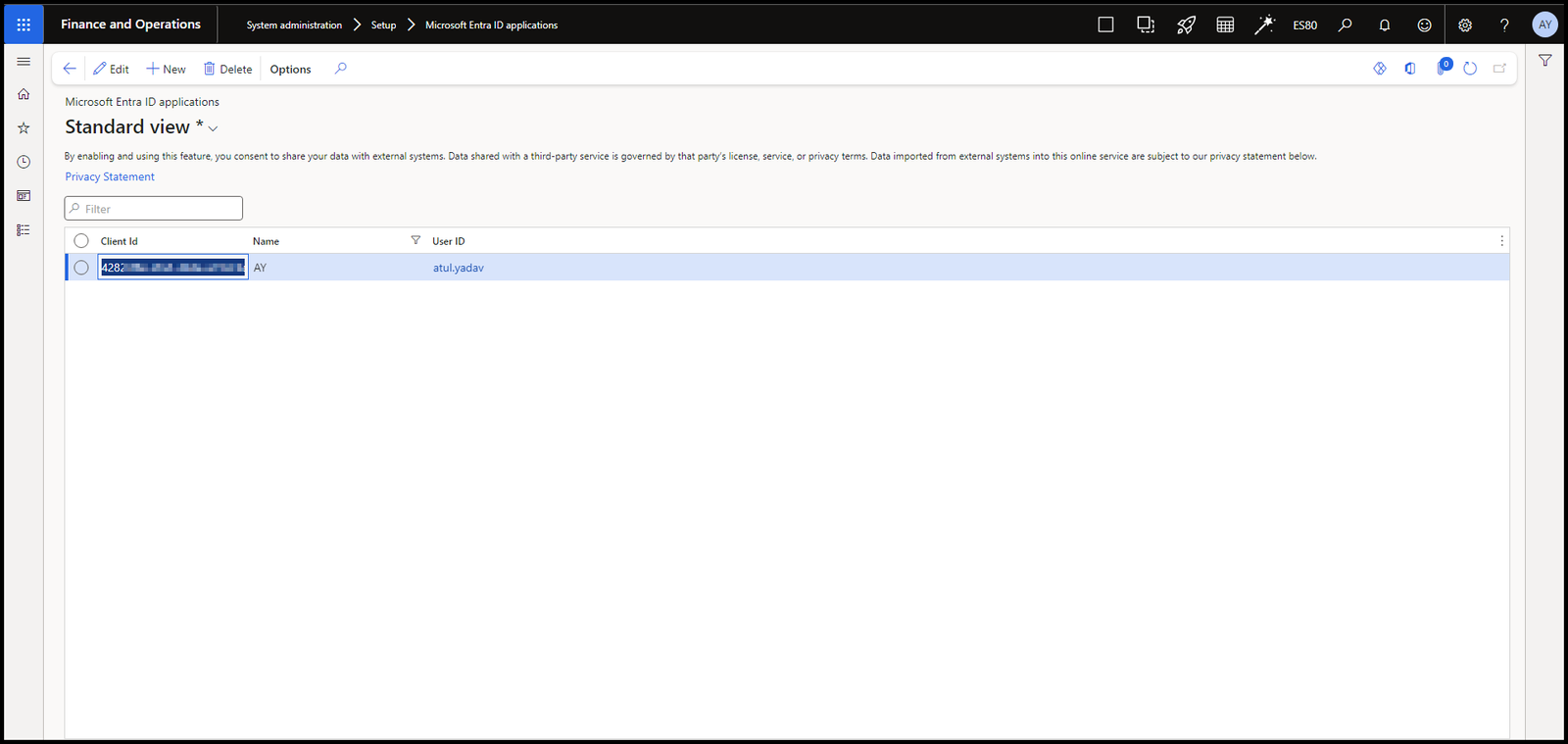
Once you have all the required data, now open Postman, you will see a screen like the one below
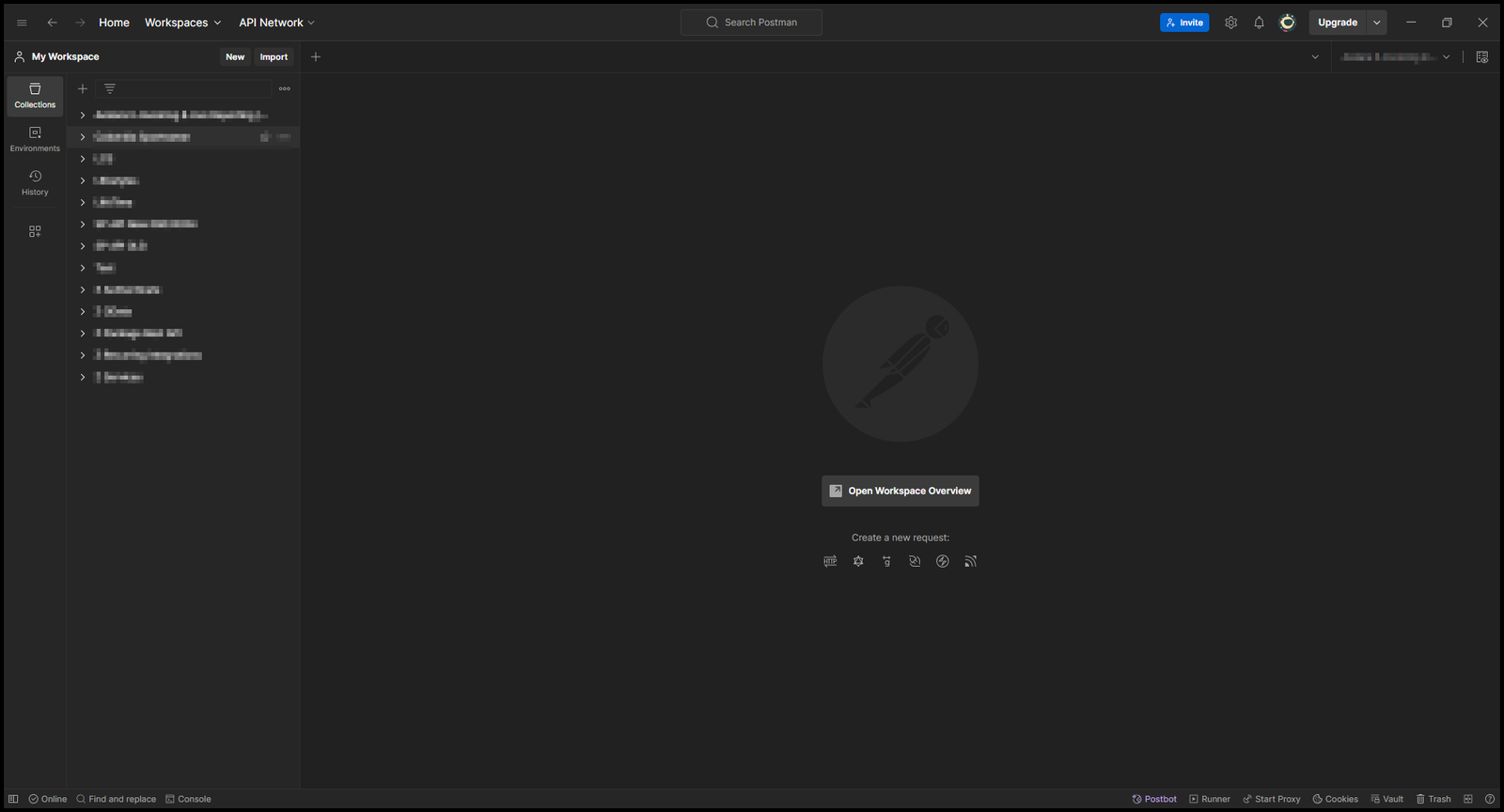
Click on New to create a new request, select HTTP as shown below
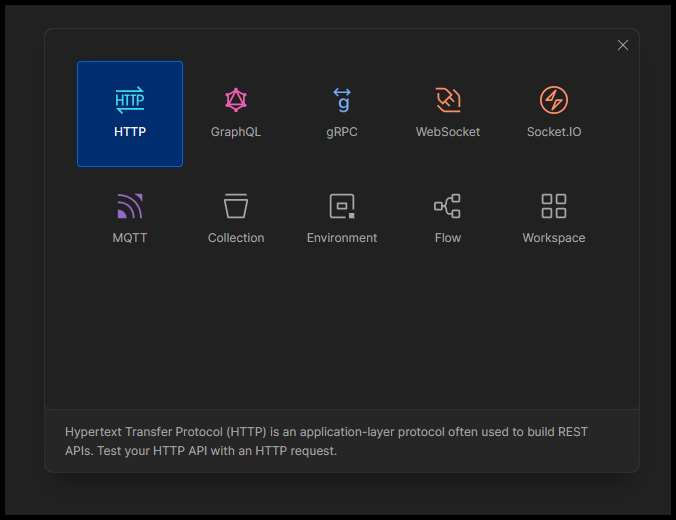
A new request will open in the URL
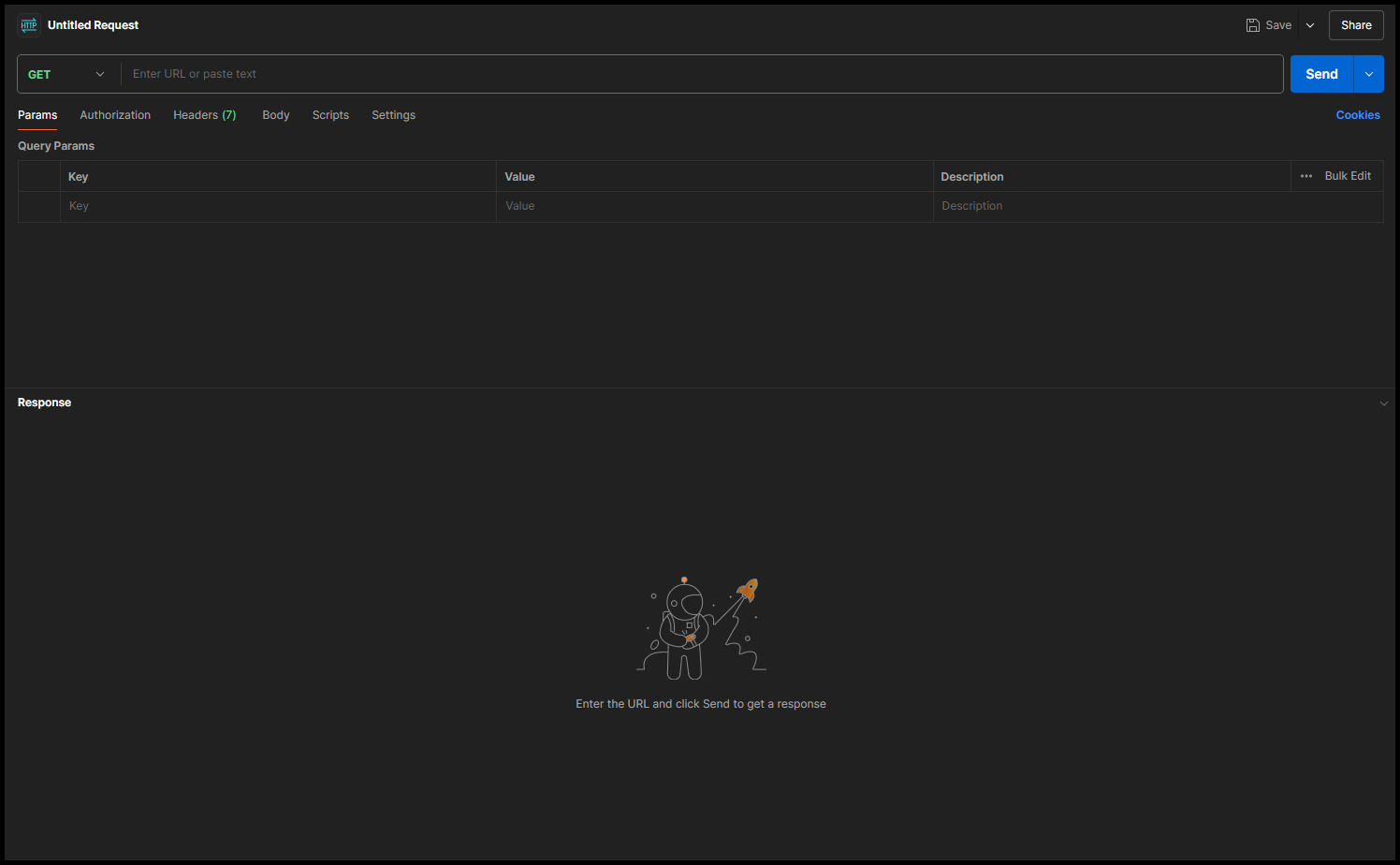
Now fill in the data as shown in the below screenshot to setup postman to call D365 custom service
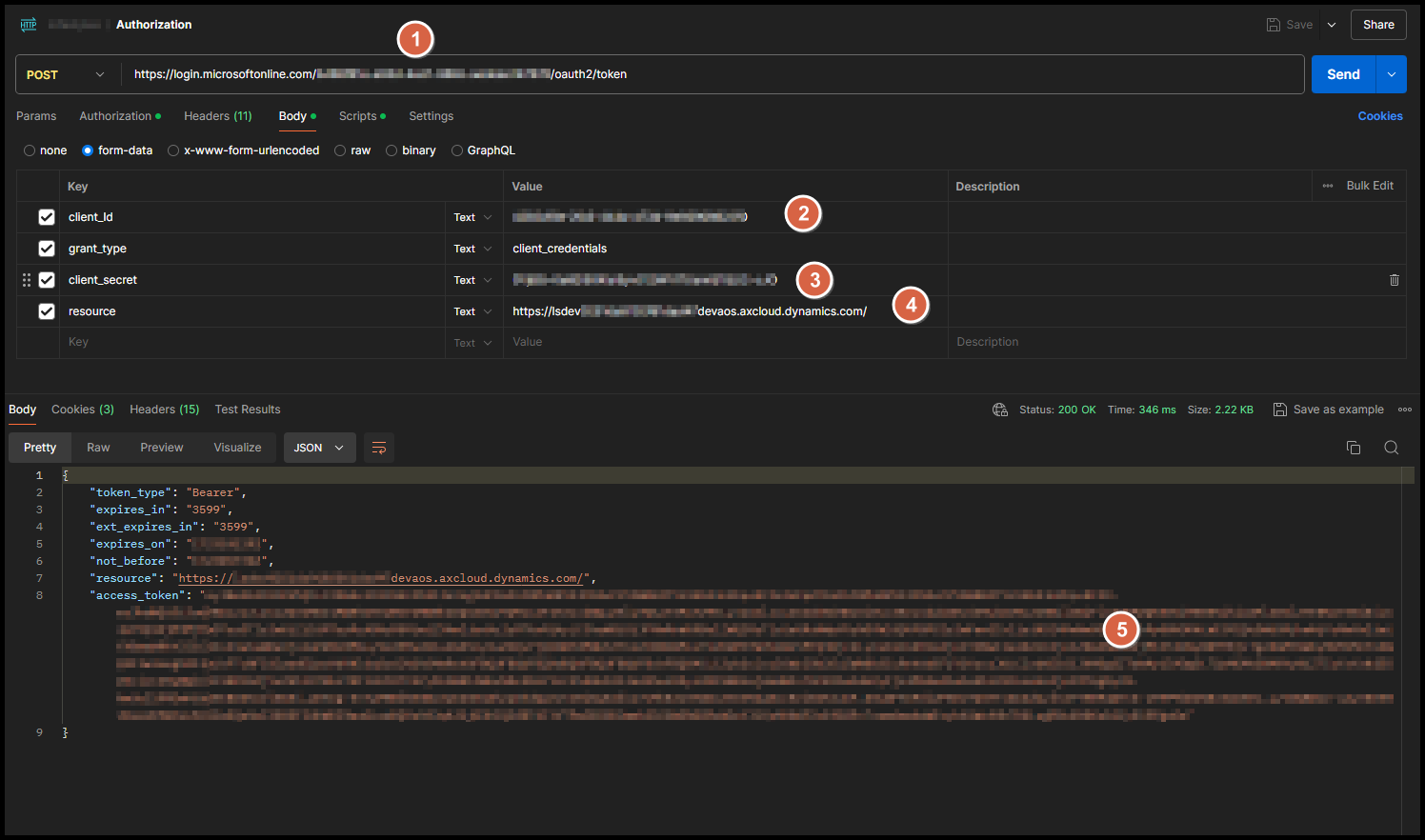
In the above screenshot
1. Tenant Id
2. Client Id
3. Client secret
4. Environment URL
5. access_token
Once all the first four fields are filled just click on Send and you will get the response, and in the response you will get the access token.
Now create a new request and in the URL put in the below URL
https://DEVURLdevaos.axcloud.dynamics.com/api/services/DCServiceGroup/DCService/create
and in the Authorization tab, put in the auth type as Bearer Token, and in the value field put in the token generated in the previous request
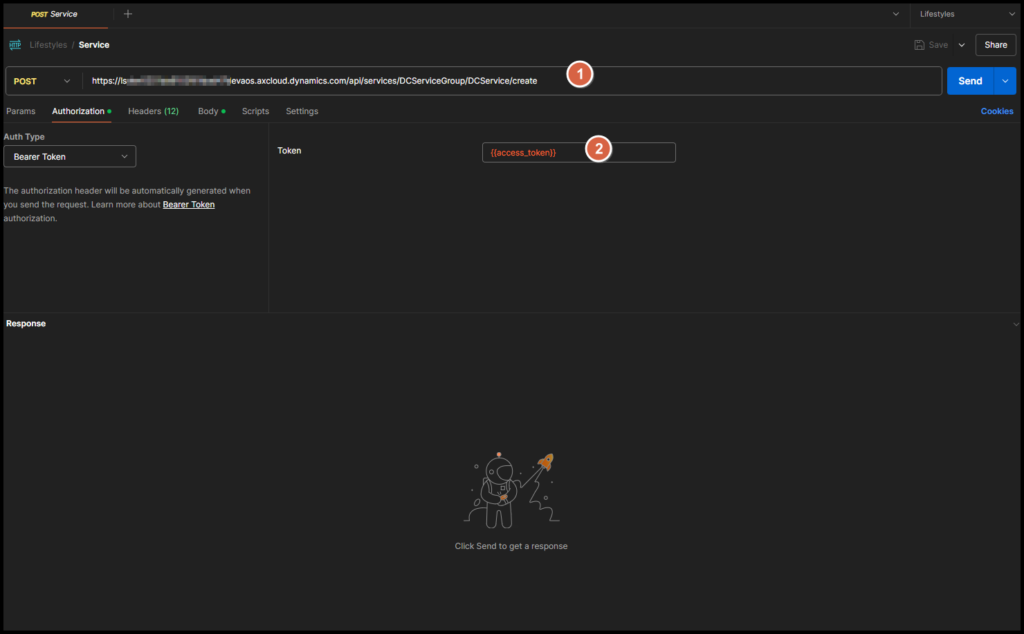
1. Request URL
2. access_token
Now go to the Body Tab and pass the below payload and click send
{
"_request" :
{
"salesId": "SO10-000000001"
}
}
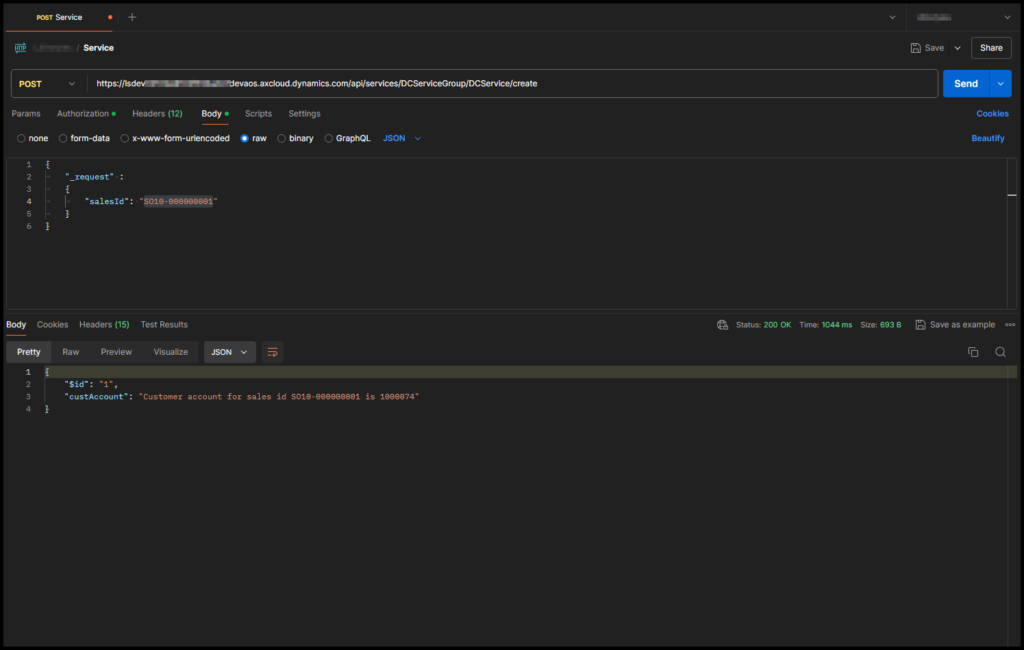
As you can see in the above picture, response is
{
"$id": "1",
"custAccount": "Customer account for sales id SO10-000000001 is 1000074"
}
The above example was about how to Create a custom service in d365 FO, as per the requirement you can add more parameters and exceptional handling as well, and send the response accordingly.
You can also see another way of Integration in D365: Setup postman to call D365 data entities
Need help? Connect Atul
- D365 Data entitiy Insert method COC - October 30, 2024
- D365 Joins - October 16, 2024
- D365 Find method and Exist method - October 9, 2024
great work …
please share blog about multiple lists in contract class
Thank you Hamza. I will be sharing a post soon on Custom service in d365 FO with multiple lists.
Thanks Atul for the nice blog.
I have few queries —
suppose the api – https://URL/api/services/service_group_name/service_name/operation_name
for example — https://DEVURLdevaos.axcloud.dynamics.com/api/services/DCServiceGroup/DCService/create
is worked perfectly and return the response correct in postman as you mentioned in the blogs.
My query is —
1. can i share the above API to the 3rd party application?
2. if yes, then what are the approaches to send the API to 3rd party.
3. how they will consume it.
4. If in the 3rd party application, if they use CRUD operation on the shared API. How it will impact on D365FO
Kindly elaborate please.
thanks in advance!
Thank you for the kind words! I’m glad you liked it.
Regarding your queries,
1. Yes
2. Create a Postman collection export it and share it with 3rd party that is the best way.
3. Depends on which language they are using.
4. If they perform CRUD operations, it will execute in D365.(If you are using OData entity CRUD operations)
Please find OData link for your reference – https://dynamicscommunity101.com/odata-in-microsoft-dynamics-365-fo/
Thanks, Atul for the response.
Point no 2 – Kindly share me the link for the postman collection and export process.
thanks in advance!
Hi Arpan,
Once you follow the steps as shown in the blog, 2 API requests will be created, which will be in a collection, just click on 3 dots on the collection name and there you will see the export option.
Postman collection export process : https://www.youtube.com/watch?v=BWofbshDneQ
Thanks Atul for the response
Hi, I am getting this error even though I have used correct Token
{
“Message”: “Authentication failed.”,
“StackTrace”: null,
“ExceptionType”: “System.InvalidOperationException”
}
What are the possible errors
Hello Swami, were you able to solve it? I’m getting the same error